JQuery란?
HTML의 요소들을 조작하는, 편리한 Javascript를 미리 작성해둔 것. 라이브러리입니다!
- Javascript로도 모든 기능(예 - 버튼 글씨 바꾸기 등)을 구현할 수는 있지만, 1) 코드가 복잡하고, 2) 브라우저 간 호환성 문제도 고려해야 해서, jQuery라는 라이브러리가 등장하게 되었답니다.
- JQuery는 Bootstrap과 같이 Javascript와 다른 특별한 소프트웨어가 아니라 미리 작성된 Javascript 코드입니다. 전문 개발자들이 짜둔 코드를 잘 가져와서 사용하는 것임을 기억해주세요! (그렇게 때문에, 쓰기 전에 "임포트"를 해야 합니다!)
1) JQueury 사용하기
우선 JQuery를 사용하기 위해서는 JQuery CDN을 import 해주어야 합니다.
<head>태그 사이에 JQuery CDN 코드를 넣어주어야 하는데, 아래 링크를 참고하시면 됩니다!
jQuery Get Started
W3Schools offers free online tutorials, references and exercises in all the major languages of the web. Covering popular subjects like HTML, CSS, JavaScript, Python, SQL, Java, and many, many more.
www.w3schools.com
JQuery 활용하기
1) input 박스의 value 활용하기
1. 우선 Bootstrap을 사용해 간단한 postbox를 만들어 보겠습니다.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<link href="https://cdn.jsdelivr.net/npm/bootstrap@5.0.2/dist/css/bootstrap.min.css" rel="stylesheet"
integrity="sha384-EVSTQN3/azprG1Anm3QDgpJLIm9Nao0Yz1ztcQTwFspd3yD65VohhpuuCOmLASjC" crossorigin="anonymous">
<script src="https://cdn.jsdelivr.net/npm/bootstrap@5.0.2/dist/js/bootstrap.bundle.min.js"
integrity="sha384-MrcW6ZMFYlzcLA8Nl+NtUVF0sA7MsXsP1UyJoMp4YLEuNSfAP+JcXn/tWtIaxVXM"
crossorigin="anonymous"></script>
<title>자바스크립트 연습!</title>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.6.0/jquery.min.js"></script>
<style>
.mypost {
max-width: 500px;
width: 95%;
margin: 20px auto 0 auto;
box-shadow: 0px 0px 3px 0px gray;
padding: 20px;
}
.mybtn {
display: flex;
flex-direction: row;
justify-content: center;
align-items: center;
margin: 20px auto 0px auto;
}
.mybtn > button {
margin-right: 10px;
}
</style>
</head>
<body>
<div class="mypost" id="postbox">
<div class="form-floating">
<input class="form-control" placeholder="입력하세요" id="floatingTextarea"></input>
<label for="floatingTextarea">코멘트</label>
</div>
<div class="mybtn">
<button type="button" class="btn btn-dark">기록하기</button>
<button type="button" class="btn btn-outline-dark">닫기</button>
</div>
</div>
</body>
</html>
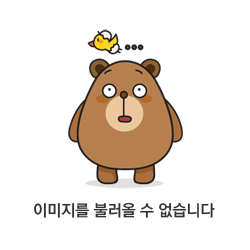
2. 이렇게 만들었으면 우선 console창에서 input 박스 안에 값을 입력해 보겠습니다.
//console창에 아래와 같이 작성해 실행시켜보겠습니다.
$('#floatingTextarea').val('이렇게 입력해 보겠습니다!')
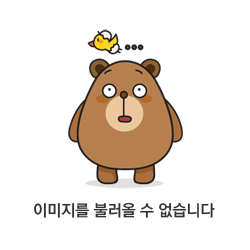
위와 같이 input박스에 입력하고자 한 value가 입력되는 것을 볼 수 있습니다!
3. 반대로 input박스에 입력된 값을 가져와 보겠습니다.
값을 가져오는 방법은 입력하는 것보다 간단합니다.
//아래와 같이 코드를 입력하면 됩니다!
$('#floatingTextarea').val()
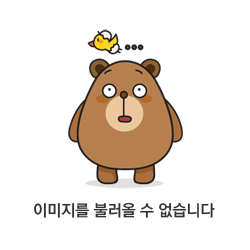
2) div 보이기/숨기기
1. 우선 보이게 하는 버튼을 하나 만들어 줍니다.
<body>
<div class="mybtn">
<button type="button" class="btn btn-dark">열기</button>
</div>
<div class="mypost" id="postbox">
<div class="form-floating">
<input class="form-control" placeholder="입력하세요" id="floatingTextarea"></input>
<label for="floatingTextarea">코멘트</label>
</div>
<div class="mybtn">
<button type="button" class="btn btn-dark">기록하기</button>
<button type="button" class="btn btn-outline-dark">닫기</button>
</div>
</div>
</body>
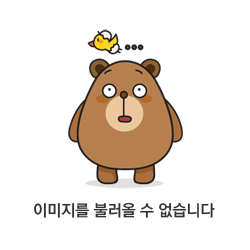
2. <script>태그 안에 post박스를 열고 닫는 함수를 정의해 줍니다.
<script>
function open_box() {
$('#postbox').show() //postbox를 보여주는 JQuery코드
}
function close_box() {
$('#postbox').hide() //postbox를 숨겨주는 JQuery코드
}
</script>
3. 버튼에 onclick 이벤트를 넣어줍니다.
//보여주는 버튼
<button type="button" class="btn btn-dark" onclick="open_box()">열기</button>
//숨기는 버튼
<button type="button" class="btn btn-outline-dark" onclick="close_box()">닫기</button>
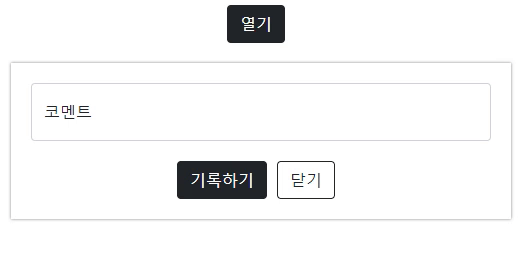
처음부터 postbox가 나와있어 닫기부터 눌러줘야하는 번거로움이 있습니다.
이 점을 보완하기 위해서 postbox에 display : none; 을 주면 해결됩니다!!
3. 태그 내 HTML 입력하기.
1. <div>태그 내에 동적으로 HTML을 넣고 싶을 때는 <div>태그에 id를 추가해 줍니다.
<div class="mycomment" id="comment">
<li>코멘트입니다.</li>
</div>
2. 함수를 작성해 줍니다.
<script>
function upload() {
let newcomment = $('#floatingTextarea').val(); //input박스에 적힌 값을 저장할 변수
let temp_html = `<li>${newcomment}</li>`; //`(백틱)을 사용하면 문자 중간에 javascript 변수를 삽입할 수 있음
$('#comment').append(temp_html); //div태그에 추가
}
</script>
3. 마지막으로 버튼에 onclick 이벤트를 넣어줍니다.
<button type="button" class="btn btn-dark" onclick="upload()">기록하기</button>
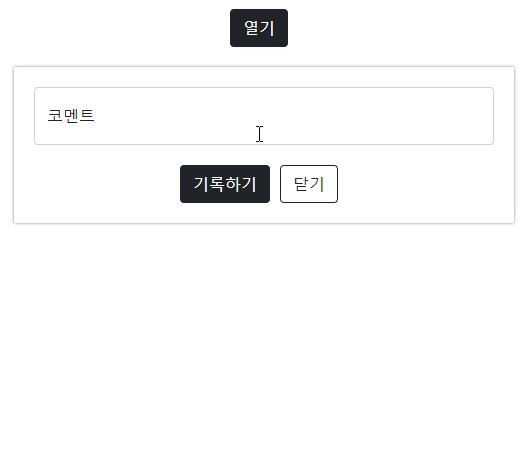
전체코드입니다!!
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<link href="https://cdn.jsdelivr.net/npm/bootstrap@5.0.2/dist/css/bootstrap.min.css" rel="stylesheet"
integrity="sha384-EVSTQN3/azprG1Anm3QDgpJLIm9Nao0Yz1ztcQTwFspd3yD65VohhpuuCOmLASjC" crossorigin="anonymous">
<script src="https://cdn.jsdelivr.net/npm/bootstrap@5.0.2/dist/js/bootstrap.bundle.min.js"
integrity="sha384-MrcW6ZMFYlzcLA8Nl+NtUVF0sA7MsXsP1UyJoMp4YLEuNSfAP+JcXn/tWtIaxVXM"
crossorigin="anonymous"></script>
<title>자바스크립트 연습!</title>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.6.0/jquery.min.js"></script>
<style>
.mypost {
max-width: 500px;
width: 95%;
margin: 20px auto 0 auto;
box-shadow: 0px 0px 3px 0px gray;
padding: 20px;
display: none;
}
.mybtn {
display: flex;
flex-direction: row;
justify-content: center;
align-items: center;
margin: 20px auto 0px auto;
}
.mybtn > button {
margin-right: 10px;
}
.mycomment{
display: flex;
flex-direction: column;
justify-content: center;
align-items: center;
margin: 40px auto 0px auto;
}
</style>
<script>
function open_box() {
$('#postbox').show()
}
function close_box() {
$('#postbox').hide()
}
function upload() {
let newcomment = $('#floatingTextarea').val();
let temp_html = `<li>${newcomment}</li>`;
$('#comment').append(temp_html);
}
</script>
</head>
<body>
<div class="mybtn">
<button type="button" class="btn btn-dark" onclick="open_box()">열기</button>
</div>
<div class="mypost" id="postbox">
<div class="form-floating">
<input class="form-control" placeholder="입력하세요" id="floatingTextarea"></input>
<label for="floatingTextarea">코멘트</label>
</div>
<div class="mybtn">
<button type="button" class="btn btn-dark" onclick="upload()">기록하기</button>
<button type="button" class="btn btn-outline-dark" onclick="close_box()">닫기</button>
</div>
</div>
<div class="mycomment" id="comment">
</div>
</body>
</html>
'SpartaCodingClub > 사전캠프' 카테고리의 다른 글
[스파르타코딩클럽] 사전캠프 6일차 - python & crawling(크롤링) (0) | 2022.10.22 |
---|---|
[스파르타코딩클럽] 사전캠프 5일차 - Ajax (0) | 2022.10.21 |
[스파르타코딩클럽] 사전캠프 3일차 - Javascript (0) | 2022.10.19 |
[스파르타코딩클럽] 사전캠프 2일차 - CSS & 부트스트랩 적용하기! (0) | 2022.10.18 |
[스파르타코딩클럽] 사전캠프 1일차 - 웹의 동작 개념 (0) | 2022.10.17 |
댓글